DATA Analysis
Contents
DATA Analysis¶
The purpose of this Notebook is to perform the data Analysis, that is comparing the outputs generated from the previous notebooks for all the different samples.
Packages installation (since Aug 2021)¶
#pip install -U wxPython
Libraries¶
import numpy
import math
import matplotlib
import matplotlib.pyplot as plt
from matplotlib import cm
import pandas as pd
from glob import glob
from functools import reduce
import ipywidgets as widgets
from ipywidgets import interact, interactive, fixed, interact_manual
import re
from itertools import cycle
import wx
#%matplotlib notebook
---------------------------------------------------------------------------
ModuleNotFoundError Traceback (most recent call last)
<ipython-input-1-51e0e51b5ca4> in <module>
11 import re
12 from itertools import cycle
---> 13 import wx
14
15
ModuleNotFoundError: No module named 'wx'
1. Data import and merging¶
1.1 XP-Ramp¶
spl = 'ASW'
XP_Ramp_df = pd.read_csv('..\DATA\DATA-Processing\PAC\XP_list_test.csv')
XP_Ramp_df_I = XP_Ramp_df.set_index('Date')
XP_Ramp_df_I.head(5)
Sample | 20K | 25K | 30K | 40K | 50K | 55K | 60K | 65K | 70K | ... | 137K | 138K | 140K | 142K | 145K | 150K | 155K | 160K | 180K | 200K | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Date | |||||||||||||||||||||
2020_09_15 | ASW | [1] | NaN | NaN | NaN | NaN | NaN | NaN | NaN | NaN | ... | NaN | NaN | [3] | NaN | NaN | [4] | NaN | NaN | NaN | NaN |
2020_09_16 | ASW | [1, 2] | NaN | NaN | [3, 4, 5] | NaN | NaN | [6, 7, 8] | NaN | NaN | ... | NaN | NaN | [21, 22, 23] | NaN | NaN | [24, 25, 26] | NaN | NaN | [27, 28, 29] | [30, 31] |
2020_09_17 | ASW | [1, 2] | NaN | NaN | [3, 4, 5] | NaN | NaN | [6, 7, 8] | NaN | NaN | ... | NaN | NaN | [21, 22, 23] | NaN | NaN | [24, 25, 26] | NaN | NaN | [27, 28] | NaN |
2020_09_21 | ASW | [1, 2] | NaN | NaN | [3, 4, 5] | NaN | NaN | [6, 7, 8] | NaN | NaN | ... | NaN | NaN | [21, 22, 23] | NaN | NaN | [24, 25, 26] | NaN | NaN | [27, 28] | NaN |
2020_09_28 | ASW | [1, 2] | NaN | [3, 4, 5, 6] | [7, 8, 9, 10] | [11, 12, 13, 14] | NaN | [15, 16, 17, 18] | NaN | [19, 20, 21, 22] | ... | NaN | NaN | [59, 60, 61, 62] | NaN | [63, 64, 65, 66] | [67, 68, 69, 70, 71, 72, 73, 74] | [75, 76, 77, 78] | [79, 80] | NaN | NaN |
5 rows × 31 columns
1.2 DR1 - DR2 - DR3 all dates¶
DR1 - DR2 - DR3 are merged together
1.2.1 Import¶
#DR1
DR1_Allscans = glob('..\DATA\DATA-Processing\PAC\XP_1-1/Samples/*/Data/DR/DR1_*_All-scans.csv')
# DR2
DR2_Allscans = glob('..\DATA\DATA-Processing\PAC\XP_1-1/Samples/*/Data/DR/DR2_*_All-scans.csv')
# DR3
DR3_Allscans = glob("..\DATA\DATA-Processing\PAC\XP_1-1/Samples/*\Data\DR\DR3_*_A.csv")
Sanity Check¶
DR1_Allscans
['..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2020_09_15\\Data\\DR\\DR1_2020_09_15_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2020_09_16\\Data\\DR\\DR1_2020_09_16_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2020_09_17\\Data\\DR\\DR1_2020_09_17_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2020_09_21\\Data\\DR\\DR1_2020_09_21_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2020_09_28\\Data\\DR\\DR1_2020_09_28_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2020_10_14\\Data\\DR\\DR1_2020_10_14_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2020_10_22\\Data\\DR\\DR1_2020_10_22_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2020_11_16\\Data\\DR\\DR1_2020_11_16_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2020_11_19\\Data\\DR\\DR1_2020_11_19_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2020_11_23\\Data\\DR\\DR1_2020_11_23_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2021_05_13\\Data\\DR\\DR1_2021_05_13_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2021_05_24\\Data\\DR\\DR1_2021_05_24_All-scans.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1/Samples\\2021_07_13\\Data\\DR\\DR1_2021_07_13_All-scans.csv']
#DR2_Allscans
#DR3_Allscans
1.2.2 Merging¶
DR1¶
All_data_frame = []
for items in DR1_Allscans:
df = pd.read_csv(items)
df_1 = df.T.iloc[1:].T
All_data_frame.append(df_1)
del df
del df_1
DR1_Allscans_full = reduce(lambda left,right: pd.merge(left,right, on=['Wavenumber'],how='outer'), All_data_frame)
DR1_Allscans_full
Wavenumber | ASW_2020_09_15_1 | ASW_2020_09_15_2 | ASW_2020_09_15_3 | ASW_2020_09_15_4 | ASW_2020_09_16_1 | ASW_2020_09_16_2 | ASW_2020_09_16_3 | ASW_2020_09_16_4 | ASW_2020_09_16_5 | ... | ASW_2021_07_13_17 | ASW_2021_07_13_18 | ASW_2021_07_13_19 | ASW_2021_07_13_20 | ASW_2021_07_13_21 | ASW_2021_07_13_22 | ASW_2021_07_13_23 | ASW_2021_07_13_24 | ASW_2021_07_13_25 | ASW_2021_07_13_26 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 799.8442 | 0.070200 | 0.086859 | 0.080537 | 0.055492 | 0.068765 | 0.070958 | 0.079536 | 0.080457 | 0.083105 | ... | 0.112301 | 0.112165 | 0.111290 | 0.114546 | 0.111352 | 0.112160 | 0.114865 | 0.117401 | 0.118798 | 0.112614 |
1 | 800.3264 | 0.069613 | 0.085440 | 0.084133 | 0.045511 | 0.066157 | 0.068055 | 0.073433 | 0.073509 | 0.076351 | ... | 0.101721 | 0.100925 | 0.100979 | 0.103694 | 0.101778 | 0.100835 | 0.104572 | 0.107281 | 0.107575 | 0.111304 |
2 | 800.8085 | 0.069002 | 0.081987 | 0.086396 | 0.034734 | 0.063596 | 0.065323 | 0.067591 | 0.067142 | 0.069968 | ... | 0.090586 | 0.090152 | 0.090122 | 0.092359 | 0.091307 | 0.089453 | 0.093991 | 0.096408 | 0.096146 | 0.109683 |
3 | 801.2906 | 0.069099 | 0.078084 | 0.083552 | 0.036551 | 0.063784 | 0.066162 | 0.069236 | 0.069118 | 0.071792 | ... | 0.089263 | 0.090480 | 0.089502 | 0.090657 | 0.089994 | 0.088894 | 0.093375 | 0.095749 | 0.095276 | 0.107832 |
4 | 801.7727 | 0.068733 | 0.076453 | 0.081117 | 0.039316 | 0.064503 | 0.067363 | 0.070878 | 0.071425 | 0.073453 | ... | 0.094123 | 0.094984 | 0.094239 | 0.095002 | 0.094698 | 0.094322 | 0.098065 | 0.100489 | 0.100310 | 0.107623 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
6634 | 3998.2570 | -0.017155 | -0.016794 | -0.016967 | -0.006420 | -0.017952 | -0.016452 | -0.014667 | -0.014350 | -0.013844 | ... | -0.019303 | -0.018898 | -0.018461 | -0.015902 | -0.016764 | -0.017420 | -0.018147 | -0.019271 | -0.019650 | -0.019098 |
6635 | 3998.7390 | -0.017126 | -0.017087 | -0.017292 | -0.006350 | -0.018364 | -0.016865 | -0.014762 | -0.014372 | -0.013879 | ... | -0.019276 | -0.018882 | -0.018438 | -0.015903 | -0.016772 | -0.017392 | -0.018119 | -0.019247 | -0.019640 | -0.019025 |
6636 | 3999.2210 | -0.017211 | -0.017489 | -0.017849 | -0.006541 | -0.018844 | -0.017376 | -0.015208 | -0.014730 | -0.014253 | ... | -0.019218 | -0.018809 | -0.018348 | -0.015880 | -0.016743 | -0.017337 | -0.018042 | -0.019167 | -0.019563 | -0.018901 |
6637 | 3999.7030 | -0.017211 | -0.016742 | -0.017107 | -0.006789 | -0.018146 | -0.016685 | -0.014652 | -0.014254 | -0.013813 | ... | -0.019852 | -0.019407 | -0.018991 | -0.016492 | -0.017368 | -0.018002 | -0.018668 | -0.019787 | -0.020182 | -0.019602 |
6638 | 4000.1850 | -0.017199 | -0.016014 | -0.016281 | -0.006947 | -0.017547 | -0.016091 | -0.014062 | -0.013732 | -0.013332 | ... | -0.020471 | -0.019999 | -0.019634 | -0.017090 | -0.017998 | -0.018664 | -0.019310 | -0.020400 | -0.020810 | -0.020218 |
6639 rows × 2041 columns
#DR1_Allscans_full.to_csv('..\DATA\DATA-Processing\PAC\DR1_full.csv')
DR2¶
All_data_frame = []
for items in DR2_Allscans:
df = pd.read_csv(items)
df_1 = df.T.iloc[1:].T
All_data_frame.append(df_1)
del df
del df_1
DR2_Allscans_full = reduce(lambda left,right: pd.merge(left,right, on=['Wavenumber'],how='outer'), All_data_frame)
#DR2_Allscans_full.to_csv('D:\DATA-Processing\PAC\DR2_full.csv')
DR2_Allscans_full
#DR2_Allscans = glob("D:\PhD-WS\Projects\PAC\XP_1-1\DATA\*\Data\DR\DR2*All-scans.csv")
Wavenumber | ASW_2020_09_15_1 | ASW_2020_09_15_2 | ASW_2020_09_15_3 | ASW_2020_09_15_4 | ASW_2020_09_16_1 | ASW_2020_09_16_2 | ASW_2020_09_16_3 | ASW_2020_09_16_4 | ASW_2020_09_16_5 | ... | ASW_2021_07_13_17 | ASW_2021_07_13_18 | ASW_2021_07_13_19 | ASW_2021_07_13_20 | ASW_2021_07_13_21 | ASW_2021_07_13_22 | ASW_2021_07_13_23 | ASW_2021_07_13_24 | ASW_2021_07_13_25 | ASW_2021_07_13_26 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 799.8442 | 0.060549 | 0.076188 | 0.071446 | 0.047759 | 0.061813 | 0.062677 | 0.070377 | 0.071031 | 0.073033 | ... | 0.093934 | 0.093203 | 0.092399 | 0.099772 | 0.096810 | 0.097544 | 0.100107 | 0.103579 | 0.104936 | 0.101043 |
1 | 800.3264 | 0.059965 | 0.074772 | 0.075044 | 0.037780 | 0.059208 | 0.059777 | 0.064277 | 0.064086 | 0.066281 | ... | 0.083360 | 0.081969 | 0.082094 | 0.088924 | 0.087240 | 0.086223 | 0.089819 | 0.093463 | 0.093718 | 0.099738 |
2 | 800.8085 | 0.059358 | 0.071323 | 0.077309 | 0.027005 | 0.056651 | 0.057048 | 0.058439 | 0.057722 | 0.059902 | ... | 0.072232 | 0.071203 | 0.071244 | 0.077594 | 0.076774 | 0.074845 | 0.079243 | 0.082596 | 0.082294 | 0.098120 |
3 | 801.2906 | 0.059458 | 0.067423 | 0.074469 | 0.028824 | 0.056841 | 0.057890 | 0.060087 | 0.059701 | 0.061729 | ... | 0.070915 | 0.071538 | 0.070631 | 0.075896 | 0.075466 | 0.074291 | 0.078632 | 0.081942 | 0.081429 | 0.096272 |
4 | 801.7727 | 0.059095 | 0.065795 | 0.072036 | 0.031592 | 0.057564 | 0.059094 | 0.061732 | 0.062012 | 0.063394 | ... | 0.075783 | 0.076050 | 0.075375 | 0.080245 | 0.080174 | 0.079724 | 0.083327 | 0.086687 | 0.086468 | 0.096067 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
6634 | 3998.2570 | 0.000151 | 0.000688 | 0.000876 | 0.000556 | 0.000884 | 0.000916 | 0.000533 | 0.000411 | 0.000489 | ... | 0.001156 | 0.001089 | 0.001162 | 0.001180 | 0.001225 | 0.001234 | 0.001153 | 0.001119 | 0.001150 | 0.001113 |
6635 | 3998.7390 | 0.000183 | 0.000399 | 0.000554 | 0.000626 | 0.000476 | 0.000507 | 0.000442 | 0.000392 | 0.000458 | ... | 0.001186 | 0.001108 | 0.001187 | 0.001181 | 0.001220 | 0.001265 | 0.001183 | 0.001146 | 0.001162 | 0.001189 |
6636 | 3999.2210 | 0.000101 | 0.000000 | 0.000000 | 0.000436 | 0.000000 | 0.000000 | 0.000000 | 0.000039 | 0.000088 | ... | 0.001247 | 0.001184 | 0.001280 | 0.001206 | 0.001251 | 0.001322 | 0.001264 | 0.001229 | 0.001242 | 0.001314 |
6637 | 3999.7030 | 0.000105 | 0.000750 | 0.000746 | 0.000190 | 0.000703 | 0.000696 | 0.000560 | 0.000518 | 0.000532 | ... | 0.000616 | 0.000588 | 0.000641 | 0.000596 | 0.000628 | 0.000660 | 0.000640 | 0.000611 | 0.000625 | 0.000614 |
6638 | 4000.1850 | 0.000120 | 0.001481 | 0.001575 | 0.000033 | 0.001306 | 0.001295 | 0.001154 | 0.001045 | 0.001017 | ... | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 |
6639 rows × 1876 columns
DR2_Allscans_full
Wavenumber | ASW_2020_09_15_1 | ASW_2020_09_15_2 | ASW_2020_09_15_3 | ASW_2020_09_15_4 | ASW_2020_09_16_1 | ASW_2020_09_16_2 | ASW_2020_09_16_3 | ASW_2020_09_16_4 | ASW_2020_09_16_5 | ... | ASW_2021_07_13_17 | ASW_2021_07_13_18 | ASW_2021_07_13_19 | ASW_2021_07_13_20 | ASW_2021_07_13_21 | ASW_2021_07_13_22 | ASW_2021_07_13_23 | ASW_2021_07_13_24 | ASW_2021_07_13_25 | ASW_2021_07_13_26 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 799.8442 | 0.060549 | 0.076188 | 0.071446 | 0.047759 | 0.061813 | 0.062677 | 0.070377 | 0.071031 | 0.073033 | ... | 0.093934 | 0.093203 | 0.092399 | 0.099772 | 0.096810 | 0.097544 | 0.100107 | 0.103579 | 0.104936 | 0.101043 |
1 | 800.3264 | 0.059965 | 0.074772 | 0.075044 | 0.037780 | 0.059208 | 0.059777 | 0.064277 | 0.064086 | 0.066281 | ... | 0.083360 | 0.081969 | 0.082094 | 0.088924 | 0.087240 | 0.086223 | 0.089819 | 0.093463 | 0.093718 | 0.099738 |
2 | 800.8085 | 0.059358 | 0.071323 | 0.077309 | 0.027005 | 0.056651 | 0.057048 | 0.058439 | 0.057722 | 0.059902 | ... | 0.072232 | 0.071203 | 0.071244 | 0.077594 | 0.076774 | 0.074845 | 0.079243 | 0.082596 | 0.082294 | 0.098120 |
3 | 801.2906 | 0.059458 | 0.067423 | 0.074469 | 0.028824 | 0.056841 | 0.057890 | 0.060087 | 0.059701 | 0.061729 | ... | 0.070915 | 0.071538 | 0.070631 | 0.075896 | 0.075466 | 0.074291 | 0.078632 | 0.081942 | 0.081429 | 0.096272 |
4 | 801.7727 | 0.059095 | 0.065795 | 0.072036 | 0.031592 | 0.057564 | 0.059094 | 0.061732 | 0.062012 | 0.063394 | ... | 0.075783 | 0.076050 | 0.075375 | 0.080245 | 0.080174 | 0.079724 | 0.083327 | 0.086687 | 0.086468 | 0.096067 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
6634 | 3998.2570 | 0.000151 | 0.000688 | 0.000876 | 0.000556 | 0.000884 | 0.000916 | 0.000533 | 0.000411 | 0.000489 | ... | 0.001156 | 0.001089 | 0.001162 | 0.001180 | 0.001225 | 0.001234 | 0.001153 | 0.001119 | 0.001150 | 0.001113 |
6635 | 3998.7390 | 0.000183 | 0.000399 | 0.000554 | 0.000626 | 0.000476 | 0.000507 | 0.000442 | 0.000392 | 0.000458 | ... | 0.001186 | 0.001108 | 0.001187 | 0.001181 | 0.001220 | 0.001265 | 0.001183 | 0.001146 | 0.001162 | 0.001189 |
6636 | 3999.2210 | 0.000101 | 0.000000 | 0.000000 | 0.000436 | 0.000000 | 0.000000 | 0.000000 | 0.000039 | 0.000088 | ... | 0.001247 | 0.001184 | 0.001280 | 0.001206 | 0.001251 | 0.001322 | 0.001264 | 0.001229 | 0.001242 | 0.001314 |
6637 | 3999.7030 | 0.000105 | 0.000750 | 0.000746 | 0.000190 | 0.000703 | 0.000696 | 0.000560 | 0.000518 | 0.000532 | ... | 0.000616 | 0.000588 | 0.000641 | 0.000596 | 0.000628 | 0.000660 | 0.000640 | 0.000611 | 0.000625 | 0.000614 |
6638 | 4000.1850 | 0.000120 | 0.001481 | 0.001575 | 0.000033 | 0.001306 | 0.001295 | 0.001154 | 0.001045 | 0.001017 | ... | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 |
6639 rows × 1876 columns
print(DR2_Allscans_full['Wavenumber'])
0 799.8442
1 800.3264
2 800.8085
3 801.2906
4 801.7727
...
6634 3998.2570
6635 3998.7390
6636 3999.2210
6637 3999.7030
6638 4000.1850
Name: Wavenumber, Length: 6639, dtype: float64
\(\color{red}{\text{Problem with Row number (should be 6639)!}}\)
DR3¶
All_data_frame = []
#Wavenumber =
for items in DR3_Allscans:
df = pd.read_csv(items)
df_1 = df.T.iloc[1:].T
All_data_frame.append(df_1)
DR3_Allscans_full = reduce(lambda left,right: pd.merge(left,right, on=['Wavenumber'],how='outer'), All_data_frame)
DR3_Allscans_full
Wavenumber | ASW_2020_09_15_1 | ASW_2020_09_15_2 | ASW_2020_09_15_3 | ASW_2020_09_15_4 | ASW_2020_09_16_1 | ASW_2020_09_16_2 | ASW_2020_09_16_3 | ASW_2020_09_16_4 | ASW_2020_09_16_5 | ... | ASW_2021_07_13_17 | ASW_2021_07_13_18 | ASW_2021_07_13_19 | ASW_2021_07_13_20 | ASW_2021_07_13_21 | ASW_2021_07_13_22 | ASW_2021_07_13_23 | ASW_2021_07_13_24 | ASW_2021_07_13_25 | ASW_2021_07_13_26 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | 2800.178 | -0.000187 | 0.000075 | 0.000050 | 9.007620e-19 | -0.000259 | -0.000271 | -0.000180 | -0.000149 | -0.000381 | ... | 0.000134 | 0.000101 | 0.000235 | 0.000275 | 0.000239 | 0.000220 | 0.000243 | 0.000233 | 0.000244 | 0.001587 |
1 | 2800.660 | -0.000137 | 0.000142 | 0.000364 | 3.145895e-04 | -0.000121 | -0.000137 | 0.000113 | 0.000146 | -0.000087 | ... | 0.000020 | -0.000026 | 0.000106 | 0.000145 | 0.000109 | 0.000093 | 0.000105 | 0.000098 | 0.000109 | 0.001548 |
2 | 2801.142 | -0.000201 | -0.000020 | 0.000000 | 9.304404e-05 | -0.000172 | -0.000191 | -0.000143 | -0.000134 | -0.000362 | ... | 0.000304 | 0.000252 | 0.000380 | 0.000414 | 0.000382 | 0.000361 | 0.000364 | 0.000374 | 0.000384 | 0.001655 |
3 | 2801.624 | -0.000130 | 0.000175 | 0.000488 | 4.249901e-04 | -0.000048 | -0.000069 | 0.000192 | 0.000211 | -0.000008 | ... | 0.000201 | 0.000159 | 0.000272 | 0.000309 | 0.000273 | 0.000247 | 0.000250 | 0.000267 | 0.000272 | 0.001589 |
4 | 2802.106 | -0.000186 | 0.000049 | 0.000172 | 2.575800e-04 | -0.000066 | -0.000087 | -0.000006 | -0.000010 | -0.000224 | ... | 0.000465 | 0.000433 | 0.000532 | 0.000573 | 0.000525 | 0.000505 | 0.000505 | 0.000521 | 0.000534 | 0.001676 |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
2485 | 3998.257 | 0.000156 | 0.000714 | 0.000909 | 5.772753e-04 | 0.000921 | 0.000955 | 0.000556 | 0.000428 | 0.000510 | ... | 0.001123 | 0.001057 | 0.001128 | 0.001146 | 0.001190 | 0.001198 | 0.001120 | 0.001087 | 0.001117 | 0.001081 |
2486 | 3998.739 | 0.000190 | 0.000414 | 0.000575 | 6.505353e-04 | 0.000497 | 0.000529 | 0.000461 | 0.000409 | 0.000478 | ... | 0.001152 | 0.001075 | 0.001153 | 0.001147 | 0.001184 | 0.001228 | 0.001149 | 0.001113 | 0.001129 | 0.001154 |
2487 | 3999.221 | 0.000105 | 0.000000 | 0.000000 | 4.531809e-04 | 0.000000 | 0.000000 | 0.000000 | 0.000040 | 0.000092 | ... | 0.001211 | 0.001150 | 0.001243 | 0.001171 | 0.001214 | 0.001283 | 0.001227 | 0.001193 | 0.001207 | 0.001276 |
2488 | 3999.703 | 0.000109 | 0.000779 | 0.000774 | 1.974239e-04 | 0.000733 | 0.000726 | 0.000584 | 0.000540 | 0.000554 | ... | 0.000598 | 0.000571 | 0.000622 | 0.000579 | 0.000609 | 0.000641 | 0.000622 | 0.000593 | 0.000607 | 0.000596 |
2489 | 4000.185 | 0.000125 | 0.001538 | 0.001636 | 3.402556e-05 | 0.001362 | 0.001350 | 0.001203 | 0.001089 | 0.001061 | ... | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 | 0.000000 |
2490 rows × 1853 columns
Row number –> OK :) (2490)
#DR3_Allscans_full.to_csv('D:\DATA-Processing\PAC\DR3_full.csv')
1.3 Data_annex¶
data_anex = glob("..\DATA\DATA-Processing\PAC\XP_1-1\Samples\*\Data\DR\**_data_annex.csv")
data_anex
['..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2020_09_15\\Data\\DR\\2020_09_15_data_annex.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2020_09_16\\Data\\DR\\2020_09_16_data_annex.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2020_09_17\\Data\\DR\\2020_09_17_data_annex.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2020_09_21\\Data\\DR\\2020_09_21_data_annex.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2020_09_28\\Data\\DR\\2020_09_28_data_annex.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2020_10_22\\Data\\DR\\2020_10_22_data_annex.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2020_11_16\\Data\\DR\\2020_11_16_data_annex.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2020_11_19\\Data\\DR\\2020_11_19_data_annex.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2020_11_23\\Data\\DR\\2020_11_23_data_annex.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2021_05_13\\Data\\DR\\2021_05_13_data_annex.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2021_05_24\\Data\\DR\\2021_05_24_data_annex.csv',
'..\\DATA\\DATA-Processing\\PAC\\XP_1-1\\Samples\\2021_07_13\\Data\\DR\\2021_07_13_data_annex.csv']
1.2.2 Merging¶
concat, not append
All_data_frame = []
for items in data_anex:
df = pd.read_csv(items)
#print(df)
#df_1 = df.T.iloc[1:].T
All_data_frame.append(df)
print(All_data_frame)
#del df
#del df_1
data_anex_full = pd.concat(All_data_frame)
[ Name min1 index1 min2 index2 min3 index3 \
0 ASW_2020_09_15_1 -0.017293 6630 -0.009476 4293 -0.009151 4147
1 ASW_2020_09_15_2 -0.017489 6636 -0.008995 4161 -0.009001 4132
2 ASW_2020_09_15_3 -0.017849 6636 -0.009715 4151 -0.009664 4132
3 ASW_2020_09_15_4 -0.006971 6630 -0.004298 4149 -0.004222 4120
min4 index4 min5 ... maxA0w maxBi maxB maxBw \
0 0.001203 2482 0.001967 ... 3270.249 2936 0.008422 2215.361
1 0.002430 2480 0.003482 ... 3246.143 2976 0.012062 2234.646
2 0.001595 2463 0.003063 ... 3233.125 2974 0.012331 2233.682
3 0.002220 2473 0.002968 ... 3228.304 2976 0.006884 2234.646
maxCi maxC maxCw Int_A Int_C Int_N_A
0 1777 0.022221 1656.579 96.292003 6.703636 100.000000
1 1754 0.021662 1645.490 111.470194 7.622334 115.762670
2 1691 0.021226 1615.116 112.359014 8.424437 116.685717
3 1574 0.013023 1558.708 53.700867 4.010565 55.768771
[4 rows x 31 columns], Name min1 index1 min2 index2 min3 index3 \
0 ASW_2020_09_16_1 -0.018844 6636 -0.008464 4235 -0.008369 4139
1 ASW_2020_09_16_2 -0.017376 6636 -0.006804 4235 -0.006714 4139
2 ASW_2020_09_16_3 -0.015208 6636 -0.005247 4197 -0.005165 4130
3 ASW_2020_09_16_4 -0.014749 6631 -0.004931 4197 -0.004867 4130
4 ASW_2020_09_16_5 -0.014274 6620 -0.004424 4245 -0.004362 4130
5 ASW_2020_09_16_6 -0.013616 6636 -0.003845 4235 -0.003754 4139
6 ASW_2020_09_16_7 -0.013178 6632 -0.003492 4235 -0.003383 4139
7 ASW_2020_09_16_8 -0.012907 6632 -0.003190 4235 -0.003086 4139
8 ASW_2020_09_16_9 -0.013285 6636 -0.002969 4245 -0.002883 4139
9 ASW_2020_09_16_10 -0.013301 6636 -0.002779 4216 -0.002666 4139
10 ASW_2020_09_16_11 -0.013018 6636 -0.002502 4197 -0.002379 4120
11 ASW_2020_09_16_12 -0.012113 6636 -0.002024 4149 -0.001986 4130
12 ASW_2020_09_16_13 -0.011900 6636 -0.001360 4151 -0.001341 4132
13 ASW_2020_09_16_14 -0.011673 6636 -0.001157 4151 -0.001115 4130
14 ASW_2020_09_16_15 -0.011645 6636 -0.001251 4149 -0.001226 4139
15 ASW_2020_09_16_16 -0.011338 6636 -0.000905 4168 -0.000901 4139
16 ASW_2020_09_16_17 -0.011244 6636 -0.000821 4149 -0.000807 4139
17 ASW_2020_09_16_18 -0.011332 6636 -0.000989 4149 -0.001025 4130
18 ASW_2020_09_16_19 -0.010872 6636 -0.000882 4158 -0.000914 4130
19 ASW_2020_09_16_20 -0.010809 6636 -0.000910 4149 -0.000942 4130
20 ASW_2020_09_16_21 -0.011159 6636 -0.001222 4151 -0.001287 4103
21 ASW_2020_09_16_22 -0.010915 6630 -0.001349 4151 -0.001461 4111
22 ASW_2020_09_16_23 -0.010836 6630 -0.001540 4151 -0.001650 4084
23 ASW_2020_09_16_24 -0.014164 6636 -0.006225 4149 -0.006255 4101
24 ASW_2020_09_16_25 -0.013780 6636 -0.008598 4151 -0.008599 4122
25 ASW_2020_09_16_26 0.002459 6636 0.004937 4151 0.004895 4053
26 ASW_2020_09_16_27 0.007303 6636 0.007753 4533 0.008098 3959
27 ASW_2020_09_16_28 0.007890 6332 0.008135 4545 0.008503 4139
28 ASW_2020_09_16_29 0.008264 6332 0.008545 4563 0.008896 4139
29 ASW_2020_09_16_30 0.008619 6331 0.008641 4562 0.009109 4084
30 ASW_2020_09_16_31 0.008318 6636 0.008889 4543 0.009277 3959
min4 index4 min5 ... maxA0w maxBi maxB maxBw \
0 -0.001418 2403 -0.000786 ... 3268.321 2945 0.009284 2219.700
1 0.000216 2432 0.000863 ... 3264.464 2945 0.009251 2219.700
2 0.001310 2413 0.001796 ... 3264.464 2947 0.010343 2220.665
3 0.001626 2413 0.002152 ... 3264.464 2947 0.010512 2220.665
4 0.002212 2413 0.002702 ... 3264.464 2947 0.010562 2220.665
5 0.003361 2444 0.003725 ... 3255.303 2949 0.010078 2221.629
6 0.003972 2425 0.004340 ... 3255.303 2951 0.010252 2222.593
7 0.004323 2406 0.004697 ... 3255.303 2951 0.010345 2222.593
8 0.004149 2415 0.004730 ... 3251.446 2959 0.011390 2226.450
9 0.004334 2367 0.004952 ... 3251.446 2959 0.011787 2226.450
10 0.004644 2367 0.005298 ... 3250.482 2959 0.011822 2226.450
11 0.005265 2415 0.005871 ... 3251.446 2959 0.011693 2226.450
12 0.005725 2367 0.006299 ... 3250.482 2980 0.012006 2236.575
13 0.005912 2415 0.006504 ... 3250.482 2959 0.011885 2226.450
14 0.006022 2415 0.006723 ... 3250.482 2966 0.011713 2229.825
15 0.006207 2415 0.006852 ... 3247.107 2964 0.012078 2228.861
16 0.006330 2415 0.006958 ... 3247.107 2964 0.012166 2228.861
17 0.006762 2480 0.007738 ... 3246.625 2957 0.011663 2225.486
18 0.006530 2480 0.008113 ... 3246.625 2978 0.011855 2235.610
19 0.006549 2480 0.008185 ... 3246.143 2978 0.011966 2235.610
20 0.006266 2415 0.007035 ... 3242.286 2966 0.012110 2229.825
21 0.006305 2444 0.007058 ... 3232.161 2976 0.012184 2234.646
22 0.006335 2396 0.007176 ... 3228.304 2976 0.012485 2234.646
23 0.003633 2444 0.004862 ... 3228.304 2976 0.011491 2234.646
24 0.001659 2484 0.002442 ... 3228.304 2978 0.008953 2235.610
25 0.006344 2329 0.006488 ... 3227.822 2978 0.004050 2235.610
26 0.007518 2300 0.007247 ... 3228.304 2295 0.001530 1906.319
27 0.008035 2480 0.007282 ... 3242.286 2698 0.001674 2100.615
28 0.008449 2480 0.007719 ... 3242.286 2698 0.001720 2100.615
29 0.008596 2480 0.008381 ... 3228.304 2602 0.001573 2054.332
30 0.008351 2461 0.008204 ... 3223.965 2437 0.002416 1974.781
maxCi maxC maxCw Int_A Int_C Int_N_A
0 1773 0.023557 1654.651 95.913079 6.952239 100.000000
1 1771 0.023602 1653.686 96.271574 7.064048 100.373772
2 1754 0.024362 1645.490 98.372535 7.669780 102.564255
3 1754 0.024289 1645.490 98.949916 7.710412 103.166239
4 1754 0.024293 1645.490 99.128959 7.740047 103.352911
5 1756 0.023139 1646.454 102.427355 8.047927 106.791854
6 1756 0.022505 1646.454 103.570509 8.057326 107.983719
7 1756 0.022478 1646.454 103.920588 8.069566 108.348714
8 1754 0.022548 1645.490 106.316388 8.023931 110.846602
9 1754 0.022958 1645.490 107.623907 8.026164 112.209835
10 1754 0.022904 1645.490 107.980853 8.037065 112.581991
11 1754 0.020700 1645.490 108.386906 8.033410 113.005345
12 1771 0.021517 1653.686 109.276611 8.503723 113.932962
13 1771 0.021516 1653.686 109.502264 8.477687 114.168230
14 1699 0.020339 1618.973 109.378492 8.007280 114.039184
15 1769 0.020189 1652.722 109.838625 8.093852 114.518923
16 1769 0.020213 1652.722 110.041893 8.142568 114.730852
17 1754 0.021377 1645.490 109.763420 7.476483 114.440514
18 1697 0.021481 1618.009 109.666245 7.569996 114.339198
19 1697 0.021457 1618.009 109.738473 7.560006 114.414504
20 1699 0.020884 1618.973 109.665610 8.124397 114.338537
21 1535 0.020529 1539.905 109.891203 8.369954 114.573742
22 1535 0.020793 1539.905 110.395286 8.440394 115.099305
23 1697 0.019248 1618.009 108.090993 7.265889 112.696823
24 1535 0.016275 1539.905 83.840839 5.785232 87.413354
25 1535 0.009392 1539.905 27.229288 2.969525 28.389547
26 1495 0.004807 1520.620 3.170572 1.399266 3.305672
27 1495 0.006589 1520.620 2.966754 1.841489 3.093169
28 1495 0.006580 1520.620 3.023007 1.840784 3.151819
29 1574 0.004599 1558.708 3.025809 1.338555 3.154741
30 1495 0.006633 1520.620 3.256800 1.924223 3.395574
[31 rows x 31 columns], Name min1 index1 min2 index2 min3 index3 \
0 ASW_2020_09_17_1 -0.041049 6638 -0.053565 4353 -0.051591 4144
1 ASW_2020_09_17_2 -0.041270 6638 -0.053673 4355 -0.051672 4144
2 ASW_2020_09_17_3 -0.041100 6638 -0.053635 4372 -0.051417 4148
3 ASW_2020_09_17_4 -0.041266 6633 -0.053916 4361 -0.051628 4148
4 ASW_2020_09_17_5 -0.041279 6633 -0.053935 4374 -0.051636 4148
5 ASW_2020_09_17_6 -0.041531 6633 -0.054154 4374 -0.051706 4144
6 ASW_2020_09_17_7 -0.041550 6633 -0.054193 4374 -0.051757 4146
7 ASW_2020_09_17_8 -0.041348 6633 -0.054032 4374 -0.051564 4146
8 ASW_2020_09_17_9 -0.041813 6638 -0.053609 4357 -0.051546 4146
9 ASW_2020_09_17_10 -0.042010 6638 -0.053469 4338 -0.051491 4146
10 ASW_2020_09_17_11 -0.041987 6638 -0.053413 4338 -0.051391 4146
11 ASW_2020_09_17_12 -0.042061 6638 -0.052986 4322 -0.051464 4148
12 ASW_2020_09_17_13 -0.042228 6638 -0.052661 4324 -0.051230 4147
13 ASW_2020_09_17_14 -0.041972 6638 -0.052482 4295 -0.051025 4147
14 ASW_2020_09_17_15 -0.041883 6638 -0.051754 4288 -0.050816 4144
15 ASW_2020_09_17_16 -0.041787 6638 -0.051383 4242 -0.050580 4144
16 ASW_2020_09_17_17 -0.041580 6638 -0.051154 4261 -0.050351 4144
17 ASW_2020_09_17_18 -0.041343 6638 -0.050699 4230 -0.050000 4142
18 ASW_2020_09_17_19 -0.040932 6633 -0.050375 4230 -0.049711 4142
19 ASW_2020_09_17_20 -0.040861 6633 -0.050217 4230 -0.049580 4144
20 ASW_2020_09_17_21 -0.040727 6633 -0.049719 4247 -0.049215 4147
21 ASW_2020_09_17_22 -0.040232 6633 -0.049096 4228 -0.048607 4140
22 ASW_2020_09_17_23 -0.039847 6633 -0.048639 4228 -0.048192 4132
23 ASW_2020_09_17_24 -0.039404 6638 -0.046864 4184 -0.046810 4136
24 ASW_2020_09_17_25 -0.034788 6638 -0.038723 4175 -0.038613 4146
25 ASW_2020_09_17_26 -0.013245 6638 -0.012364 4213 -0.012120 4144
26 ASW_2020_09_17_27 0.005091 6638 0.005379 4562 0.005726 4132
27 ASW_2020_09_17_28 0.005975 6633 0.005630 4562 0.006364 4132
min4 index4 min5 ... maxA0w maxBi maxB maxBw \
0 -0.026561 2483 -0.023261 ... 3293.391 2940 0.010735 2217.290
1 -0.026568 2483 -0.023255 ... 3289.052 2940 0.010743 2217.290
2 -0.025492 2483 -0.021956 ... 3280.374 2940 0.011222 2217.290
3 -0.025534 2483 -0.021993 ... 3279.892 2940 0.011301 2217.290
4 -0.025461 2483 -0.021957 ... 3276.035 2940 0.011437 2217.290
5 -0.025538 2473 -0.022032 ... 3265.910 2959 0.013357 2226.450
6 -0.025422 2473 -0.021894 ... 3262.053 2959 0.013781 2226.450
7 -0.025145 2473 -0.021623 ... 3262.053 2978 0.013934 2235.610
8 -0.024607 2473 -0.020855 ... 3258.196 2978 0.014325 2235.610
9 -0.024534 2473 -0.020687 ... 3257.232 2978 0.014972 2235.610
10 -0.024377 2473 -0.020547 ... 3257.232 2978 0.015035 2235.610
11 -0.024290 2473 -0.020396 ... 3256.268 2978 0.015104 2235.610
12 -0.024098 2482 -0.020072 ... 3256.268 2988 0.015436 2240.432
13 -0.023904 2482 -0.019835 ... 3256.268 2988 0.015455 2240.432
14 -0.023720 2473 -0.019773 ... 3254.339 2988 0.015600 2240.432
15 -0.023597 2473 -0.019453 ... 3253.375 2988 0.015976 2240.432
16 -0.023371 2482 -0.019255 ... 3252.411 2988 0.015818 2240.432
17 -0.023107 2483 -0.019587 ... 3254.339 2978 0.015517 2235.610
18 -0.022701 2483 -0.019206 ... 3253.375 2978 0.015520 2235.610
19 -0.022563 2483 -0.019068 ... 3252.411 2978 0.015529 2235.610
20 -0.022598 2473 -0.018500 ... 3252.893 2988 0.015668 2240.432
21 -0.022263 2473 -0.017958 ... 3244.214 2988 0.015724 2240.432
22 -0.021806 2473 -0.017448 ... 3238.911 2988 0.015824 2240.432
23 -0.020812 2473 -0.016158 ... 3232.644 3007 0.015618 2249.592
24 -0.015231 2473 -0.011197 ... 3230.233 3026 0.013833 2258.752
25 -0.001047 2473 0.000863 ... 3228.304 3026 0.007350 2258.752
26 0.006629 2425 0.006986 ... 3224.929 2353 0.001953 1934.283
27 0.006884 2329 0.007150 ... 3241.322 2305 0.002652 1911.141
maxCi maxC maxCw Int_A Int_C Int_N_A
0 1835 0.030168 1684.542 135.148307 5.806995 100.000000
1 1835 0.030455 1684.542 135.402168 6.249885 100.187839
2 1814 0.028092 1674.418 139.668744 5.387251 103.344797
3 1814 0.027993 1674.418 140.633365 5.855341 104.058547
4 1814 0.027857 1674.418 141.038119 5.758875 104.358036
5 1814 0.028235 1674.418 146.782680 7.007985 108.608597
6 1814 0.027795 1674.418 148.618855 7.174062 109.967234
7 1814 0.027677 1674.418 149.121309 7.204706 110.339014
8 1814 0.024760 1674.418 152.408599 6.885394 112.771371
9 1814 0.024572 1674.418 154.066032 7.388391 113.997752
10 1814 0.024441 1674.418 154.444547 7.414897 114.277826
11 1776 0.024353 1656.097 155.071749 7.140653 114.741910
12 1776 0.024087 1656.097 156.217955 7.391837 115.590020
13 1776 0.024028 1656.097 156.422587 7.409158 115.741432
14 1795 0.024562 1665.257 156.144672 7.645946 115.535796
15 1835 0.023701 1684.542 156.649678 6.152382 115.909464
16 1835 0.023294 1684.542 156.939959 5.738137 116.124251
17 1814 0.023472 1674.418 156.481455 6.948400 115.784991
18 1754 0.024173 1645.490 156.748946 7.562134 115.982915
19 1754 0.024207 1645.490 156.973342 7.568792 116.148952
20 1795 0.024614 1665.257 156.551748 7.727592 115.837003
21 1776 0.024433 1656.097 156.905620 7.734879 116.098842
22 1776 0.024010 1656.097 156.991053 7.448137 116.162056
23 1697 0.022184 1618.009 156.348744 6.354408 115.686794
24 1699 0.019466 1618.973 128.467096 5.824587 95.056385
25 1697 0.011157 1618.009 55.115755 3.628102 40.781684
26 1697 0.003724 1618.009 3.576484 1.192419 2.646340
27 578 0.004862 1078.512 2.706240 1.708501 2.002422
[28 rows x 31 columns], Name min1 index1 min2 index2 min3 index3 \
0 ASW_2020_09_21_1 -0.024190 6635 -0.021363 4408 -0.019372 4147
1 ASW_2020_09_21_2 -0.023390 6635 -0.020446 4408 -0.018472 4147
2 ASW_2020_09_21_3 -0.022978 6638 -0.019493 4413 -0.017551 4145
3 ASW_2020_09_21_4 -0.022961 6638 -0.019394 4404 -0.017429 4145
4 ASW_2020_09_21_5 -0.022826 6638 -0.019274 4404 -0.017250 4145
5 ASW_2020_09_21_6 -0.022259 6638 -0.018912 4389 -0.016856 4147
6 ASW_2020_09_21_7 -0.022216 6638 -0.018716 4389 -0.016632 4147
7 ASW_2020_09_21_8 -0.022156 6638 -0.018649 4408 -0.016515 4147
8 ASW_2020_09_21_9 -0.021014 6635 -0.018046 4389 -0.016279 4147
9 ASW_2020_09_21_10 -0.020738 6635 -0.017794 4381 -0.015959 4141
10 ASW_2020_09_21_11 -0.020528 6635 -0.017625 4383 -0.015784 4141
11 ASW_2020_09_21_12 -0.020188 6634 -0.016946 4358 -0.015520 4145
12 ASW_2020_09_21_13 -0.019821 6636 -0.016477 4329 -0.015199 4145
13 ASW_2020_09_21_14 -0.019420 6635 -0.016119 4329 -0.014845 4145
14 ASW_2020_09_21_15 -0.019226 6635 -0.015382 4283 -0.014569 4147
15 ASW_2020_09_21_16 -0.018965 6638 -0.014864 4283 -0.014110 4147
16 ASW_2020_09_21_17 -0.018702 6638 -0.014561 4283 -0.013823 4147
17 ASW_2020_09_21_18 -0.018787 6638 -0.014501 4262 -0.013889 4147
18 ASW_2020_09_21_19 -0.019221 6638 -0.014377 4243 -0.013883 4147
19 ASW_2020_09_21_20 -0.019109 6638 -0.014228 4264 -0.013762 4147
20 ASW_2020_09_21_21 -0.019282 6638 -0.014379 4233 -0.013907 4145
21 ASW_2020_09_21_22 -0.019843 6638 -0.014471 4243 -0.014056 4145
22 ASW_2020_09_21_23 -0.019504 6638 -0.014153 4214 -0.013797 4145
23 ASW_2020_09_21_24 -0.018905 6638 -0.014180 4195 -0.014076 4147
24 ASW_2020_09_21_25 -0.008469 6634 -0.004991 4191 -0.004949 4141
25 ASW_2020_09_21_26 0.005774 6634 0.006492 4250 0.006626 4034
26 ASW_2020_09_21_27 0.006858 6334 0.006554 4559 0.007121 4147
27 ASW_2020_09_21_28 0.006282 6638 0.006698 4550 0.007404 4099
min4 index4 min5 ... maxA0w maxBi maxB maxBw \
0 -0.004675 2482 -0.003455 ... 3277.481 2947 0.007965 2220.665
1 -0.003923 2482 -0.002717 ... 3273.624 2947 0.007976 2220.665
2 -0.003091 2489 -0.001809 ... 3272.660 2964 0.008282 2228.861
3 -0.002985 2489 -0.001640 ... 3268.803 2964 0.008400 2228.861
4 -0.002768 2489 -0.001449 ... 3267.839 2964 0.008481 2228.861
5 -0.002739 2480 -0.001496 ... 3259.642 2974 0.009784 2233.682
6 -0.002465 2480 -0.001256 ... 3259.642 2974 0.009957 2233.682
7 -0.002311 2480 -0.001079 ... 3258.678 2974 0.010071 2233.682
8 -0.001858 2480 -0.000408 ... 3254.821 2964 0.010422 2228.861
9 -0.001698 2480 -0.000284 ... 3253.857 2974 0.010848 2233.682
10 -0.001495 2480 -0.000022 ... 3253.857 2974 0.010910 2233.682
11 -0.000897 2480 0.000702 ... 3254.339 2974 0.010826 2233.682
12 -0.000607 2480 0.000983 ... 3250.482 2974 0.011229 2233.682
13 -0.000280 2480 0.001307 ... 3250.482 2974 0.011235 2233.682
14 -0.000096 2480 0.001628 ... 3253.857 2974 0.011074 2233.682
15 0.000031 2480 0.001786 ... 3249.518 2976 0.011291 2234.646
16 0.000334 2480 0.002021 ... 3249.518 2976 0.011190 2234.646
17 0.000887 2482 0.001990 ... 3249.518 2964 0.011057 2228.861
18 0.001161 2489 0.002158 ... 3249.518 2993 0.011005 2242.842
19 0.001315 2489 0.002354 ... 3249.518 2993 0.011012 2242.842
20 0.000304 2480 0.002248 ... 3249.518 2985 0.011111 2238.985
21 0.000039 2480 0.001931 ... 3243.732 2983 0.011126 2238.021
22 0.000187 2480 0.002116 ... 3235.536 2983 0.011147 2238.021
23 -0.000267 2480 0.001992 ... 3230.715 2993 0.010732 2242.842
24 0.003089 2480 0.004251 ... 3227.340 2993 0.007173 2242.842
25 0.007346 2365 0.007449 ... 3226.376 2320 0.002181 1918.373
26 0.007820 2365 0.007820 ... 3235.536 2320 0.001697 1918.373
27 0.008061 2298 0.007917 ... 3235.536 2322 0.001954 1919.337
maxCi maxC maxCw Int_A Int_C Int_N_A
0 1785 0.019938 1660.436 88.680361 5.302705 100.000000
1 1785 0.020090 1660.436 88.678660 5.579043 99.998081
2 1771 0.019792 1653.686 90.619014 5.523779 102.186112
3 1771 0.019782 1653.686 91.273150 5.553721 102.923746
4 1771 0.019890 1653.686 91.534144 5.623779 103.218055
5 1802 0.020415 1668.632 94.993549 6.029632 107.119036
6 1802 0.020625 1668.632 96.159751 6.759778 108.434099
7 1802 0.020583 1668.632 96.483848 6.794238 108.799566
8 1771 0.018731 1653.686 98.075058 6.683564 110.593887
9 1771 0.019553 1653.686 99.088457 6.906236 111.736641
10 1771 0.019569 1653.686 99.348481 6.934031 112.029856
11 1754 0.017284 1645.490 100.108583 5.864341 112.886982
12 1756 0.017001 1646.454 100.848724 6.205336 113.721599
13 1756 0.016985 1646.454 101.084908 6.179590 113.987929
14 1754 0.018376 1645.490 101.152267 6.829697 114.063886
15 1697 0.018297 1618.009 101.661938 6.951543 114.638616
16 1697 0.018379 1618.009 101.918675 6.966613 114.928124
17 1771 0.018300 1653.686 101.998480 5.952818 115.018115
18 1771 0.018923 1653.686 102.635762 6.192575 115.736743
19 1771 0.018730 1653.686 102.776710 6.146912 115.895683
20 1754 0.018467 1645.490 102.469812 6.359011 115.549610
21 1697 0.018545 1618.009 102.715825 6.870105 115.827026
22 1697 0.018414 1618.009 102.436553 6.913507 115.512106
23 1754 0.016847 1645.490 98.934311 6.228148 111.562819
24 1570 0.011939 1556.779 60.646382 4.024592 68.387613
25 1570 0.006015 1556.779 8.738037 2.052757 9.853407
26 566 0.003866 1072.727 3.421708 1.361210 3.858473
27 1570 0.005483 1556.779 3.997098 1.954863 4.507309
[28 rows x 31 columns], Name min1 index1 min2 index2 min3 index3 \
0 ASW_2020_09_28_1 -0.026504 6620 -0.024226 4401 -0.022407 4134
1 ASW_2020_09_28_2 -0.025427 6636 -0.022822 4372 -0.021184 4144
2 ASW_2020_09_28_3 -0.024675 6636 -0.021948 4401 -0.020278 4142
3 ASW_2020_09_28_4 -0.024353 6632 -0.021656 4393 -0.019961 4142
4 ASW_2020_09_28_5 -0.024163 6632 -0.021448 4401 -0.019691 4134
.. ... ... ... ... ... ... ...
75 ASW_2020_09_28_76 0.033360 6633 0.035411 4154 0.035383 4135
76 ASW_2020_09_28_77 0.039784 6633 0.040950 4154 0.040848 4077
77 ASW_2020_09_28_78 0.043527 6335 0.043778 4163 0.043654 3962
78 ASW_2020_09_28_79 0.043639 6638 0.043704 4557 0.044042 3943
79 ASW_2020_09_28_80 0.016138 6633 0.020018 4211 0.020286 4136
min4 index4 min5 ... maxA0w maxBi maxB maxBw \
0 -0.009105 2458 -0.007995 ... 3272.178 2923 0.009211 2209.094
1 -0.007454 2477 -0.006254 ... 3276.035 2942 0.007386 2218.254
2 -0.006614 2477 -0.005435 ... 3276.035 2942 0.007681 2218.254
3 -0.006287 2477 -0.005064 ... 3271.213 2923 0.007746 2209.094
4 -0.005975 2458 -0.004768 ... 3276.035 2942 0.007936 2218.254
.. ... ... ... ... ... ... ... ...
75 0.040743 2479 0.041975 ... 3226.858 2990 0.005160 2241.396
76 0.043054 2479 0.043847 ... 3225.894 3040 0.003348 2265.502
77 0.043991 2345 0.043920 ... 3224.929 2300 0.002073 1908.730
78 0.044228 2345 0.044163 ... 3224.929 2321 0.002171 1918.855
79 0.033108 2489 0.034497 ... 3248.072 3037 0.011578 2264.056
maxCi maxC maxCw Int_A Int_C Int_N_A
0 1780 0.022529 1658.026 86.558093 6.591012 100.000000
1 1771 0.019989 1653.686 86.043652 5.563222 99.405669
2 1771 0.020173 1653.686 86.876902 5.599657 100.368318
3 1771 0.020165 1653.686 87.117734 5.607032 100.646549
4 1771 0.020096 1653.686 87.298165 5.540918 100.855000
.. ... ... ... ... ... ...
75 1675 0.008315 1607.402 40.624815 2.506664 46.933584
76 1675 0.006200 1607.402 22.869835 1.940948 26.421371
77 947 0.005038 1256.416 5.198979 2.124355 6.006347
78 947 0.005697 1256.416 3.493992 2.447063 4.036586
79 1698 0.018059 1618.491 102.387413 7.200944 118.287510
[80 rows x 31 columns], Name min1 index1 min2 index2 min3 index3 \
0 ASW_2020_10_22_1 0.014819 6636 0.018631 4326 0.019603 4134
1 ASW_2020_10_22_2 -0.022843 6636 -0.015237 4395 -0.013955 4134
2 ASW_2020_10_22_3 -0.029230 6636 -0.020667 4268 -0.019819 4143
3 ASW_2020_10_22_4 -0.027761 6636 -0.018797 4249 -0.018241 4144
4 ASW_2020_10_22_5 -0.030971 6637 -0.021733 4251 -0.021147 4134
.. ... ... ... ... ... ... ...
62 ASW_2020_10_22_63 -0.026737 6637 -0.019604 4237 -0.019420 4144
63 ASW_2020_10_22_64 -0.024439 6638 -0.017377 4237 -0.017269 4144
64 ASW_2020_10_22_65 -0.022301 6638 -0.015511 4247 -0.015393 4144
65 ASW_2020_10_22_66 -0.020372 6638 -0.013859 4245 -0.013783 4144
66 ASW_2020_10_22_67 -0.013516 6636 -0.008561 4182 -0.008546 4134
min4 index4 min5 ... maxA0w maxBi maxB maxBw \
0 0.031884 2459 0.032713 ... 3272.660 2955 0.009482 2224.521
1 0.000369 2478 0.001672 ... 3272.660 2926 0.009332 2210.540
2 -0.004564 2478 -0.003160 ... 3254.339 2974 0.010775 2233.682
3 -0.002777 2476 -0.000697 ... 3250.482 2974 0.011963 2233.682
4 -0.005513 2486 -0.003327 ... 3249.518 2974 0.011912 2233.682
.. ... ... ... ... ... ... ... ...
62 -0.006983 2469 -0.005708 ... 3226.858 2993 0.008266 2242.842
63 -0.005614 2469 -0.004496 ... 3226.376 2993 0.007831 2242.842
64 -0.004635 2459 -0.003677 ... 3226.858 3022 0.007336 2256.824
65 -0.003965 2469 -0.003115 ... 3226.376 2982 0.006928 2237.539
66 -0.002468 2486 -0.000886 ... 3226.858 2974 0.004705 2233.682
maxCi maxC maxCw Int_A Int_C Int_N_A
0 1789 0.021203 1662.365 96.288989 4.550228 100.000000
1 1789 0.022469 1662.365 95.908370 5.544767 99.604712
2 1760 0.019923 1648.383 105.914224 7.060409 109.996195
3 1760 0.019266 1648.383 108.987482 6.933768 113.187898
4 1760 0.019564 1648.383 109.252891 7.234991 113.463536
.. ... ... ... ... ... ...
62 1694 0.012402 1616.563 73.168245 5.227288 75.988175
63 1694 0.011845 1616.563 68.500794 5.015416 71.140838
64 1694 0.011156 1616.563 63.701469 4.740874 66.156546
65 1694 0.010578 1616.563 58.925336 4.483670 61.196339
66 1691 0.007734 1615.116 40.133157 2.805107 41.679902
[67 rows x 31 columns], Name min1 index1 min2 index2 min3 index3 \
0 ASW_2020_11_16_1 -0.010121 6626 -0.009355 4383 -0.008172 4147
1 ASW_2020_11_16_2 -0.026869 6637 -0.023372 4383 -0.021893 4148
2 ASW_2020_11_16_3 -0.028153 6636 -0.023769 4383 -0.022059 4147
3 ASW_2020_11_16_4 -0.026518 6636 -0.022628 4383 -0.020908 4147
4 ASW_2020_11_16_5 -0.026869 6636 -0.022787 4383 -0.020939 4147
.. ... ... ... ... ... ... ...
84 ASW_2020_11_16_85 -0.028089 6636 -0.023765 4212 -0.023512 4145
85 ASW_2020_11_16_86 -0.027660 6636 -0.023311 4224 -0.023026 4145
86 ASW_2020_11_16_87 -0.026231 6636 -0.022321 4212 -0.022064 4145
87 ASW_2020_11_16_88 -0.026257 6636 -0.022117 4212 -0.021893 4145
88 ASW_2020_11_16_89 -0.009999 6636 -0.007569 4154 -0.007616 4144
min4 index4 min5 ... maxA0w maxBi maxB maxBw \
0 0.005017 2488 0.006147 ... 3277.481 2947 0.007971 2220.665
1 -0.007076 2478 -0.005719 ... 3273.624 2928 0.008250 2211.504
2 -0.007937 2488 -0.006685 ... 3262.535 2955 0.010532 2224.521
3 -0.006668 2488 -0.005349 ... 3258.196 2955 0.010946 2224.521
4 -0.006565 2488 -0.005277 ... 3258.196 2955 0.010908 2224.521
.. ... ... ... ... ... ... ... ...
84 -0.008379 2479 -0.006823 ... 3226.376 2993 0.010435 2242.842
85 -0.008107 2488 -0.006541 ... 3226.376 2993 0.010305 2242.842
86 -0.007428 2488 -0.005895 ... 3226.376 3022 0.010178 2256.824
87 -0.007197 2479 -0.005681 ... 3226.376 2993 0.010150 2242.842
88 -0.003184 2363 -0.003027 ... 3223.001 2993 0.005525 2242.842
maxCi maxC maxCw Int_A Int_C Int_N_A
0 1790 0.021751 1662.847 96.870340 5.913422 100.000000
1 1780 0.021978 1658.026 96.939281 6.078172 100.071168
2 1779 0.022222 1657.543 103.933533 7.059747 107.291389
3 1779 0.021728 1657.543 106.204256 7.152024 109.635474
4 1779 0.021779 1657.543 106.420222 7.234453 109.858417
.. ... ... ... ... ... ...
84 1694 0.015213 1616.563 93.859992 6.298993 96.892395
85 1694 0.015076 1616.563 92.901573 6.225848 95.903012
86 1694 0.014895 1616.563 91.949100 6.146797 94.919766
87 1694 0.014744 1616.563 90.926319 6.090073 93.863941
88 1694 0.008163 1616.563 42.185939 3.502538 43.548870
[89 rows x 31 columns], Name min1 index1 min2 index2 min3 index3 \
0 ASW_2020_11_19_1 -0.022255 6636 -0.014984 4239 -0.014844 4141
1 ASW_2020_11_19_2 -0.021923 6636 -0.014678 4258 -0.014551 4141
2 ASW_2020_11_19_3 -0.020756 6636 -0.013996 4247 -0.013787 4141
3 ASW_2020_11_19_4 -0.020955 6636 -0.014144 4247 -0.013911 4141
4 ASW_2020_11_19_5 -0.020809 6636 -0.013993 4239 -0.013722 4141
.. ... ... ... ... ... ... ...
155 ASW_2020_11_19_156 -0.006684 6636 -0.001928 4166 -0.002012 4116
156 ASW_2020_11_19_157 -0.005365 6636 -0.000708 4166 -0.000795 4097
157 ASW_2020_11_19_158 0.001811 6636 0.005097 4156 0.004987 4097
158 ASW_2020_11_19_159 0.115129 6636 0.108430 4156 0.107438 3942
159 ASW_2020_11_19_160 -0.001314 6636 0.000191 4156 -0.000612 3951
min4 index4 min5 ... maxA0w maxBi maxB maxBw \
0 -0.005657 2466 -0.004707 ... 3271.696 2943 0.009633 2218.736
1 -0.005469 2466 -0.004570 ... 3267.356 2943 0.009603 2218.736
2 -0.004433 2440 -0.003640 ... 3257.714 2954 0.010272 2224.039
3 -0.004228 2440 -0.003406 ... 3257.714 2962 0.010502 2227.896
4 -0.004041 2488 -0.003245 ... 3257.714 2960 0.010316 2226.932
.. ... ... ... ... ... ... ... ...
155 0.001997 2485 0.002317 ... 3225.412 3003 0.004499 2247.664
156 0.003111 2485 0.003370 ... 3225.412 2974 0.004437 2233.682
157 0.008112 2483 0.008396 ... 3225.412 2974 0.004239 2233.682
158 0.107005 2483 0.107452 ... 3227.340 2974 0.003423 2233.682
159 -0.001645 2285 -0.002079 ... 3225.412 2934 0.002297 2214.397
maxCi maxC maxCw Int_A Int_C Int_N_A
0 1786 0.024570 1660.918 101.446420 7.401140 100.000000
1 1786 0.024570 1660.918 101.462372 7.399730 100.015724
2 1779 0.022942 1657.543 107.069815 7.809192 105.543217
3 1779 0.022413 1657.543 108.340997 7.787821 106.796275
4 1779 0.022392 1657.543 108.715090 7.893904 107.165034
.. ... ... ... ... ... ...
155 1699 0.006401 1618.973 40.564471 2.177198 39.986104
156 1757 0.006255 1646.937 39.437048 2.146906 38.874755
157 1699 0.005886 1618.973 38.505910 2.184976 37.956894
158 499 0.034891 1040.424 38.132260 3.647014 37.588572
159 1346 0.004708 1448.784 15.318774 2.090059 15.100360
[160 rows x 31 columns], Name min1 index1 min2 index2 min3 index3 \
0 ASW_2020_11_23_1 -0.048605 6636 -0.054942 4425 -0.051454 4147
1 ASW_2020_11_23_2 -0.048993 6636 -0.054901 4425 -0.051299 4147
2 ASW_2020_11_23_3 -0.048375 6636 -0.054771 4415 -0.051253 4146
3 ASW_2020_11_23_4 -0.026927 6636 -0.033931 4425 -0.030514 4146
4 ASW_2020_11_23_5 -0.047706 6636 -0.054582 4425 -0.050982 4146
.. ... ... ... ... ... ... ...
160 ASW_2020_11_23_161 -0.023476 6634 -0.021884 4557 -0.020983 4146
161 ASW_2020_11_23_162 -0.023096 6634 -0.021644 4537 -0.020652 4146
162 ASW_2020_11_23_163 -0.005374 6634 -0.003921 4547 -0.003129 4146
163 ASW_2020_11_23_164 -0.022173 6634 -0.021017 4557 -0.020003 4146
164 ASW_2020_11_23_165 -0.021724 6634 -0.020708 4555 -0.019500 4146
min4 index4 min5 ... maxA0w maxBi maxB maxBw \
0 -0.027763 2481 -0.024776 ... 3265.910 2967 0.010980 2230.307
1 -0.027272 2489 -0.024254 ... 3265.910 2967 0.010892 2230.307
2 -0.027716 2479 -0.024967 ... 3264.946 2967 0.012430 2230.307
3 -0.006862 2471 -0.004306 ... 3260.607 2967 0.013197 2230.307
4 -0.027427 2479 -0.024669 ... 3260.607 2967 0.012906 2230.307
.. ... ... ... ... ... ... ... ...
160 -0.018501 2335 -0.018258 ... 3219.626 2359 0.002775 1937.175
161 -0.018287 2335 -0.018074 ... 3218.662 2984 0.002813 2238.503
162 -0.000935 2335 -0.000807 ... 3218.662 2984 0.002908 2238.503
163 -0.017830 2335 -0.017583 ... 3218.662 2359 0.002763 1937.175
164 -0.017328 2335 -0.017110 ... 3218.662 2359 0.002770 1937.175
maxCi maxC maxCw Int_A Int_C Int_N_A
0 1801 0.022375 1668.150 119.768412 4.997663 103.317735
1 1801 0.022296 1668.150 120.179675 4.893321 103.672509
2 1809 0.023067 1672.007 122.450319 5.325348 105.631271
3 1810 0.022556 1672.489 123.960379 5.494804 106.933919
4 1810 0.023123 1672.489 124.157452 5.862579 107.103923
.. ... ... ... ... ... ...
160 588 0.005387 1083.333 6.833895 2.414610 5.895232
161 588 0.006205 1083.333 6.540537 2.772033 5.642168
162 559 0.006144 1069.352 6.359314 2.183563 5.485837
163 588 0.005447 1083.333 6.032295 2.381032 5.203735
164 559 0.005220 1069.352 5.569998 2.244073 4.804936
[165 rows x 31 columns], Name min1 index1 min2 index2 min3 index3 \
0 ASW_2021_05_13_1 -0.014722 6638 -0.004114 4151 -0.004143 4140
1 ASW_2021_05_13_2 -0.013557 6630 -0.003223 4209 -0.003271 4140
2 ASW_2021_05_13_3 -0.012140 6636 -0.002166 4153 -0.002128 4134
3 ASW_2021_05_13_4 -0.011840 6636 -0.001680 4153 -0.001689 4134
4 ASW_2021_05_13_5 -0.011791 6636 -0.001560 4153 -0.001565 4134
.. ... ... ... ... ... ... ...
842 ASW_2021_05_13_843 0.007231 6638 0.010093 4149 0.009504 3951
843 ASW_2021_05_13_844 0.008622 6638 0.010998 4151 0.010368 3951
844 ASW_2021_05_13_845 0.009758 6638 0.011605 4151 0.010888 3942
845 ASW_2021_05_13_846 0.010426 6638 0.011803 4149 0.011117 3961
846 ASW_2021_05_13_847 0.011142 6638 0.011994 4149 0.011298 3942
min4 index4 min5 ... maxA0w maxBi maxB maxBw \
0 0.002053 2392 0.002395 ... 3268.321 2928 0.008403 2211.504
1 0.002862 2392 0.003149 ... 3268.321 2926 0.008431 2210.540
2 0.003635 2390 0.003998 ... 3259.160 2953 0.009904 2223.557
3 0.004012 2447 0.004475 ... 3258.196 2943 0.010262 2218.736
4 0.004190 2388 0.004680 ... 3258.196 2972 0.010508 2232.718
.. ... ... ... ... ... ... ... ...
842 0.008941 2286 0.008657 ... 3230.233 2974 0.003747 2233.682
843 0.009187 2286 0.008756 ... 3233.125 2984 0.003207 2238.503
844 0.009167 2286 0.008658 ... 3235.054 2915 0.002778 2205.237
845 0.009026 2286 0.008403 ... 3182.021 2896 0.002373 2196.076
846 0.008909 2286 0.008190 ... 3172.860 2838 0.002158 2168.113
maxCi maxC maxCw Int_A Int_C Int_N_A
0 1790 0.021825 1662.847 90.046648 6.326076 100.000000
1 1790 0.021791 1662.847 90.114214 6.358682 100.075035
2 1780 0.020271 1658.026 94.952295 6.967246 105.447896
3 1771 0.019722 1653.686 96.240967 7.115134 106.879012
4 1781 0.019706 1658.508 96.523813 7.165569 107.193123
.. ... ... ... ... ... ...
842 1758 0.005088 1647.419 23.725832 1.953275 26.348379
843 1758 0.004406 1647.419 17.889279 1.792270 19.866680
844 1894 0.003936 1712.988 12.598864 1.622215 13.991486
845 1913 0.003616 1722.148 8.090795 1.501391 8.985115
846 1923 0.003434 1726.969 4.503201 1.384698 5.000965
[847 rows x 31 columns], Unnamed: 0 Name min1 index1 min2 index2 \
0 0 ASW_2021_05_24_1 -0.002640 6636 0.011161 4153
1 1 ASW_2021_05_24_2 -0.001645 6636 0.012008 4153
2 2 ASW_2021_05_24_3 -0.001016 6636 0.012534 4153
3 3 ASW_2021_05_24_4 0.000278 6636 0.014021 4151
4 4 ASW_2021_05_24_5 0.001221 6636 0.014551 4151
.. ... ... ... ... ... ...
487 487 ASW_2021_05_24_488 0.015455 6636 0.012918 4555
488 488 ASW_2021_05_24_489 0.015946 6636 0.013282 4555
489 489 ASW_2021_05_24_490 0.016240 6636 0.013454 4563
490 490 ASW_2021_05_24_491 0.016592 6636 0.013727 4546
491 491 ASW_2021_05_24_492 0.017505 6636 0.014293 4555
min3 index3 min4 index4 ... maxAw scan_number maxBi \
0 0.010603 3942 0.011893 2392 ... 3267.356 1 2926
1 0.011458 3942 0.012649 2363 ... 3267.356 2 2926
2 0.012014 3942 0.013173 2344 ... 3268.321 3 2926
3 0.013516 3998 0.014016 2285 ... 3259.160 4 2936
4 0.014093 3978 0.014571 2344 ... 3258.196 5 2936
.. ... ... ... ... ... ... ... ...
487 0.013973 4134 0.015475 2323 ... 3245.179 488 3241
488 0.014290 4134 0.015699 2323 ... 3245.179 489 3241
489 0.014445 4134 0.015793 2323 ... 3245.179 490 3241
490 0.014715 4134 0.016050 2342 ... 3245.179 491 3241
491 0.015249 4134 0.016378 2323 ... 3245.179 492 3244
maxB maxBw maxCi maxC maxCw Int_A Int_N_A
0 0.009988 2210.540 2 0.072921 800.8085 95.599945 100.000000
1 0.009952 2210.540 2 0.072887 800.8085 95.721654 100.127310
2 0.010010 2210.540 2 0.073617 800.8085 95.891793 100.305280
3 0.010640 2215.361 8 0.077077 803.7012 99.648824 104.235231
4 0.010740 2215.361 8 0.078190 803.7012 100.622867 105.254105
.. ... ... ... ... ... ... ...
487 0.020827 2362.409 2 0.058832 800.8085 7.858836 8.220544
488 0.020510 2362.409 2 0.060971 800.8085 7.890271 8.253426
489 0.020154 2362.409 2 0.062315 800.8085 7.835753 8.196399
490 0.019851 2362.409 2 0.063480 800.8085 7.833736 8.194289
491 0.019413 2363.855 2 0.070444 800.8085 7.880451 8.243154
[492 rows x 28 columns], Unnamed: 0 Name min1 index1 min2 index2 \
0 0 ASW_2021_07_13_1 -0.023247 6638 -0.016507 4321
1 1 ASW_2021_07_13_2 -0.024986 6638 -0.017637 4321
2 2 ASW_2021_07_13_3 -0.025060 6638 -0.017461 4311
3 3 ASW_2021_07_13_4 -0.024484 6638 -0.016808 4323
4 4 ASW_2021_07_13_5 -0.023746 6638 -0.016474 4325
5 5 ASW_2021_07_13_6 -0.023772 6638 -0.016451 4333
6 6 ASW_2021_07_13_7 -0.025788 6638 -0.017365 4246
7 7 ASW_2021_07_13_8 -0.027713 6638 -0.018953 4196
8 8 ASW_2021_07_13_9 -0.028306 6638 -0.019867 4227
9 9 ASW_2021_07_13_10 -0.028047 6638 -0.019582 4198
10 10 ASW_2021_07_13_11 -0.027592 6638 -0.019192 4198
11 11 ASW_2021_07_13_12 -0.027410 6638 -0.019096 4227
12 12 ASW_2021_07_13_13 -0.027203 6638 -0.018973 4227
13 13 ASW_2021_07_13_14 -0.026585 6638 -0.018483 4198
14 14 ASW_2021_07_13_15 -0.025243 6638 -0.017489 4179
15 15 ASW_2021_07_13_16 -0.021303 6638 -0.013459 4227
16 16 ASW_2021_07_13_17 -0.020471 6638 -0.012965 4227
17 17 ASW_2021_07_13_18 -0.019999 6638 -0.012605 4227
18 18 ASW_2021_07_13_19 -0.019634 6638 -0.012372 4198
19 19 ASW_2021_07_13_20 -0.017090 6638 -0.011731 4179
20 20 ASW_2021_07_13_21 -0.017998 6638 -0.012285 4198
21 21 ASW_2021_07_13_22 -0.018664 6638 -0.012685 4198
22 22 ASW_2021_07_13_23 -0.019310 6638 -0.013218 4198
23 23 ASW_2021_07_13_24 -0.020400 6638 -0.014330 4198
24 24 ASW_2021_07_13_25 -0.020810 6638 -0.014576 4198
25 25 ASW_2021_07_13_26 -0.020218 6638 -0.016644 4390
min3 index3 min4 index4 ... maxAw scan_number maxBi \
0 -0.015874 4148 -0.003954 2416 ... 3271.213 1 2931
1 -0.017018 4148 -0.004779 2464 ... 3274.106 2 2931
2 -0.016754 4148 -0.004488 2464 ... 3274.106 3 2931
3 -0.015983 4141 -0.003471 2464 ... 3261.089 4 2960
4 -0.015621 4141 -0.003115 2445 ... 3256.750 5 2960
5 -0.015600 4141 -0.003059 2445 ... 3256.750 6 2960
6 -0.016911 4148 -0.003640 2474 ... 3255.785 7 2960
7 -0.018817 4148 -0.005100 2436 ... 3250.964 8 2969
8 -0.019682 4129 -0.005615 2466 ... 3244.697 9 2979
9 -0.019390 4148 -0.005116 2466 ... 3241.804 10 2979
10 -0.018974 4129 -0.004603 2476 ... 3238.911 11 2979
11 -0.018885 4148 -0.004401 2485 ... 3237.947 12 2979
12 -0.018723 4148 -0.004244 2476 ... 3236.983 13 2979
13 -0.018245 4148 -0.003778 2485 ... 3236.018 14 2979
14 -0.017222 4148 -0.002918 2466 ... 3233.608 15 2979
15 -0.013217 4148 0.001125 2466 ... 3233.608 16 2979
16 -0.012714 4148 0.001476 2485 ... 3232.644 17 2979
17 -0.012382 4148 0.001704 2466 ... 3232.644 18 2979
18 -0.012103 4148 0.001858 2466 ... 3231.679 19 2998
19 -0.011503 4148 0.002747 2466 ... 3224.929 20 2998
20 -0.012054 4148 0.002371 2485 ... 3224.929 21 2998
21 -0.012469 4148 0.002138 2466 ... 3224.929 22 2998
22 -0.012967 4148 0.001726 2476 ... 3224.929 23 3236
23 -0.014095 4148 0.000553 2476 ... 3224.929 24 3236
24 -0.014322 4148 0.000491 2476 ... 3224.929 25 3236
25 -0.014663 4148 0.000497 2474 ... 3211.912 26 3236
maxB maxBw maxCi maxC maxCw Int_A Int_N_A
0 0.010150 2212.950 1 0.063002 800.3264 102.978270 100.000000
1 0.009962 2212.950 1 0.063354 800.3264 103.109427 100.127364
2 0.010023 2212.950 1 0.064041 800.3264 103.321193 100.333006
3 0.011388 2226.932 6 0.070443 802.7370 110.353134 107.161574
4 0.011925 2226.932 5 0.073464 802.2549 111.998335 108.759193
5 0.012024 2226.932 6 0.074375 802.7370 112.343960 109.094822
6 0.011853 2226.932 6 0.074133 802.7370 114.658673 111.342590
7 0.012560 2231.271 8 0.076707 803.7012 116.219512 112.858288
8 0.012491 2236.093 8 0.077200 803.7012 116.285704 112.922566
9 0.012425 2236.093 8 0.076597 803.7012 115.741609 112.394207
10 0.012335 2236.093 8 0.077850 803.7012 115.030556 111.703718
11 0.012174 2236.093 8 0.078411 803.7012 114.121406 110.820862
12 0.012126 2236.093 8 0.077994 803.7012 113.180598 109.907264
13 0.011935 2236.093 8 0.077645 803.7012 112.192297 108.947546
14 0.012013 2236.093 8 0.077545 803.7012 111.215388 107.998890
15 0.011903 2236.093 8 0.085764 803.7012 110.193054 107.006123
16 0.011663 2236.093 8 0.087491 803.7012 109.234792 106.075576
17 0.011675 2236.093 8 0.087453 803.7012 108.374365 105.240033
18 0.011615 2245.253 8 0.086546 803.7012 107.784448 104.667177
19 0.011730 2245.253 8 0.091422 803.7012 109.120932 105.965009
20 0.011549 2245.253 8 0.091772 803.7012 108.956293 105.805131
21 0.011747 2245.253 8 0.091667 803.7012 108.758884 105.613431
22 0.311595 2359.999 8 0.094514 803.7012 109.178866 106.021267
23 0.621625 2359.999 8 0.097058 803.7012 110.082086 106.898365
24 0.662658 2359.999 8 0.098187 803.7012 110.049914 106.867123
25 0.691713 2359.999 1 0.099738 800.3264 113.285420 110.009054
[26 rows x 28 columns]]
data_anex_full
Name | min1 | index1 | min2 | index2 | min3 | index3 | min4 | index4 | min5 | ... | maxBi | maxB | maxBw | maxCi | maxC | maxCw | Int_A | Int_C | Int_N_A | Unnamed: 0 | |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
0 | ASW_2020_09_15_1 | -0.017293 | 6630 | -0.009476 | 4293 | -0.009151 | 4147 | 0.001203 | 2482 | 0.001967 | ... | 2936 | 0.008422 | 2215.361 | 1777 | 0.022221 | 1656.5790 | 96.292003 | 6.703636 | 100.000000 | NaN |
1 | ASW_2020_09_15_2 | -0.017489 | 6636 | -0.008995 | 4161 | -0.009001 | 4132 | 0.002430 | 2480 | 0.003482 | ... | 2976 | 0.012062 | 2234.646 | 1754 | 0.021662 | 1645.4900 | 111.470194 | 7.622334 | 115.762670 | NaN |
2 | ASW_2020_09_15_3 | -0.017849 | 6636 | -0.009715 | 4151 | -0.009664 | 4132 | 0.001595 | 2463 | 0.003063 | ... | 2974 | 0.012331 | 2233.682 | 1691 | 0.021226 | 1615.1160 | 112.359014 | 8.424437 | 116.685717 | NaN |
3 | ASW_2020_09_15_4 | -0.006971 | 6630 | -0.004298 | 4149 | -0.004222 | 4120 | 0.002220 | 2473 | 0.002968 | ... | 2976 | 0.006884 | 2234.646 | 1574 | 0.013023 | 1558.7080 | 53.700867 | 4.010565 | 55.768771 | NaN |
0 | ASW_2020_09_16_1 | -0.018844 | 6636 | -0.008464 | 4235 | -0.008369 | 4139 | -0.001418 | 2403 | -0.000786 | ... | 2945 | 0.009284 | 2219.700 | 1773 | 0.023557 | 1654.6510 | 95.913079 | 6.952239 | 100.000000 | NaN |
... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... | ... |
21 | ASW_2021_07_13_22 | -0.018664 | 6638 | -0.012685 | 4198 | -0.012469 | 4148 | 0.002138 | 2466 | 0.004063 | ... | 2998 | 0.011747 | 2245.253 | 8 | 0.091667 | 803.7012 | 108.758884 | NaN | 105.613431 | 21.0 |
22 | ASW_2021_07_13_23 | -0.019310 | 6638 | -0.013218 | 4198 | -0.012967 | 4148 | 0.001726 | 2476 | 0.003659 | ... | 3236 | 0.311595 | 2359.999 | 8 | 0.094514 | 803.7012 | 109.178866 | NaN | 106.021267 | 22.0 |
23 | ASW_2021_07_13_24 | -0.020400 | 6638 | -0.014330 | 4198 | -0.014095 | 4148 | 0.000553 | 2476 | 0.002548 | ... | 3236 | 0.621625 | 2359.999 | 8 | 0.097058 | 803.7012 | 110.082086 | NaN | 106.898365 | 23.0 |
24 | ASW_2021_07_13_25 | -0.020810 | 6638 | -0.014576 | 4198 | -0.014322 | 4148 | 0.000491 | 2476 | 0.002494 | ... | 3236 | 0.662658 | 2359.999 | 8 | 0.098187 | 803.7012 | 110.049914 | NaN | 106.867123 | 24.0 |
25 | ASW_2021_07_13_26 | -0.020218 | 6638 | -0.016644 | 4390 | -0.014663 | 4148 | 0.000497 | 2474 | 0.003447 | ... | 3236 | 0.691713 | 2359.999 | 1 | 0.099738 | 800.3264 | 113.285420 | NaN | 110.009054 | 25.0 |
2017 rows × 32 columns
data_anex_full.to_csv('..\DATA\DATA-Processing\PAC\Data_Annex_full.csv')
2 Scans selection (Temperature - isotherms)¶
Using I python widget
2.1 Parameter list¶
Temp = XP_Ramp_df_I.columns[1:].values.tolist()
Date = XP_Ramp_df_I.index.values.tolist()
Sample = XP_Ramp_df_I['Sample'].values.tolist()
2.2 Widget selection¶
the purpose of the widget is to select the data we want to plot using widget
create list
Document:
#Create Dropdown Box Widget
wT = widgets.SelectMultiple(
options= Temp,
description='Temperature:',
disabled=False,
)
wD = widgets.SelectMultiple(
options= Date,
description='Date',
disabled=False,
)
wS = widgets.SelectMultiple(
options= Sample,
description='Sample',
disabled=False,
)
widgets.HBox([wS,wD, wT])
display(wT,wD,wS)
wT_L = list(wT.value)
wD_L = list(wD.value)
sample neme construction¶
use list value to extract scan number and produce file name
insert condition to supress first scan (ramp)
#date = ['2020_09_16','2020_09_17']
#temp = ['60K','80K']
#spl = 'ASW'
z = []
value_1 = []
for x in wD_L:
for y in wT_L:
value = XP_Ramp_df_I.loc[XP_Ramp_df_I.index == x, y].values[0]
value_1 = re.findall(r"[-+]?\d*\.\d+|\d+", value)
for items in value_1:
to_plot = str('{}_{}_{}'.format(spl, x, items))
z.append({
'Name' : str(to_plot),
'Temp' : y,
'Date' : x,
})
z
[{'Name': 'ASW_2020_09_15_1', 'Temp': '20K ', 'Date': '2020_09_15'},
{'Name': 'ASW_2020_09_16_1', 'Temp': '20K ', 'Date': '2020_09_16'},
{'Name': 'ASW_2020_09_16_2', 'Temp': '20K ', 'Date': '2020_09_16'},
{'Name': 'ASW_2020_09_17_1', 'Temp': '20K ', 'Date': '2020_09_17'},
{'Name': 'ASW_2020_09_17_2', 'Temp': '20K ', 'Date': '2020_09_17'}]
dat= pd.DataFrame(z)
data_df = dat.set_index('Name')
data_df
Temp | Date | |
---|---|---|
Name | ||
ASW_2020_09_15_1 | 20K | 2020_09_15 |
ASW_2020_09_16_1 | 20K | 2020_09_16 |
ASW_2020_09_16_2 | 20K | 2020_09_16 |
ASW_2020_09_17_1 | 20K | 2020_09_17 |
ASW_2020_09_17_2 | 20K | 2020_09_17 |
1.1.4 Plot pre-formating¶
Temperature¶
Find a way to have a specific c=cmjet when we want to plot long isotherm data.
def Temp_color(row):
if row['Temp'] == '20K ':
return int(1)
if row['Temp'] == '30K ':
return int(2)
elif row['Temp'] == '40K':
return int(3)
elif row['Temp'] == '50K':
return int(4)
elif row['Temp'] == '60K':
return int(5)
elif row['Temp'] == '70K':
return int(6)
elif row['Temp'] == '80K':
return int(7)
elif row['Temp'] == '90K':
return int(8)
elif row['Temp'] == '100K':
return int(9)
elif row['Temp'] == '110K':
return int(10)
elif row['Temp'] == '120K':
return int(11)
elif row['Temp'] == '125K':
return int(12)
elif row['Temp'] == '130K':
return int(13)
elif row['Temp'] == '132K':
return int(14)
elif row['Temp'] == '134K':
return int(15)
elif row['Temp'] == '135K':
return int(16)
elif row['Temp'] == '136K':
return int(17)
elif row['Temp'] == '137K':
return int(18)
elif row['Temp'] == '138K':
return int(19)
elif row['Temp'] == '140K':
return int(20)
elif row['Temp'] == '145K':
return int(21)
elif row['Temp'] == '150K':
return int(21)
elif row['Temp'] == '155K':
return int(22)
elif row['Temp'] == '160K':
return int(23)
elif row['Temp'] == '180K':
return int(24)
elif row['Temp'] == '200K':
return int(25)
data_df['Colour'] = data_df.apply (lambda row: Temp_color(row), axis=1)
data_df
Temp | Date | Colour | |
---|---|---|---|
Name | |||
ASW_2020_09_15_1 | 20K | 2020_09_15 | 1 |
ASW_2020_09_16_1 | 20K | 2020_09_16 | 1 |
ASW_2020_09_16_2 | 20K | 2020_09_16 | 1 |
ASW_2020_09_17_1 | 20K | 2020_09_17 | 1 |
ASW_2020_09_17_2 | 20K | 2020_09_17 | 1 |
Dates¶
linestyle = ['-',':','--']
6 sample max
LD = dict(zip(wD_L, linestyle))
LD
{'2020_09_15': '-', '2020_09_16': ':', '2020_09_17': '--'}
data_df['linestyle'] = data_df['Date'].map(LD)
data_df
Temp | Date | Colour | linestyle | |
---|---|---|---|---|
Name | ||||
ASW_2020_09_15_1 | 20K | 2020_09_15 | 1 | - |
ASW_2020_09_16_1 | 20K | 2020_09_16 | 1 | : |
ASW_2020_09_16_2 | 20K | 2020_09_16 | 1 | : |
ASW_2020_09_17_1 | 20K | 2020_09_17 | 1 | -- |
ASW_2020_09_17_2 | 20K | 2020_09_17 | 1 | -- |
For plotting
Could try Class attribute¶
What do we want to do:
Vocabulary:
Method = function associated with a class
Attributes =
Class XP_Param is a blueprint to construct a list of sample that we want to plot based on the parameters enters with the widget.
One function should find within XP_Ramp_df_I the scan numbers associated with a peculiar date and temperature. A second function should build a string with the format spl_date_scan number and append it into a list
differences between instance variables and class variables ?
Plotting¶
Purpose here is to plot from DR2_all scan the scan present in dat using Temp and date in the legend and use the groupping for formatting
Find way to implement label with respect to dataframe value (input for color ?)
3 different DR¶
print(dat['Name'])
0 ASW_2020_09_15_1
1 ASW_2020_09_16_1
2 ASW_2020_09_16_2
3 ASW_2020_09_17_1
4 ASW_2020_09_17_2
Name: Name, dtype: object
nscan = len(list(data_df['Date'].values.tolist()))
print(nscan)
fig, ax= plt.subplots(figsize=(10,10))
#colors = sns.color_palette("coolwarm", data_df.Temp.nunique())
#ax.set_prop_cycle('color', colors)
#ax.set_prop_cycle(color =['b', 'g'])
#cc = (cycler(linestyle=['-', '--', '-.', ]))
for i in dat['Name']:
#DR2
#x = DR2_Allscans_full.Wavenumber
#y = DR2_Allscans_full['{}'.format(i)]
#`DR3
x = DR3_Allscans_full.Wavenumber
y = DR3_Allscans_full['{}'.format(i)]
plt.plot(x,y, label="{}_{}".format(str(data_df.loc[i]['Date']), str(data_df.loc[i]['Temp'])), c=cm.jet(int(data_df.loc[i]['Colour'])/25), linestyle=(data_df.loc[i]['linestyle']))
#plt.title('{0} DR1 '.format(date))
plt.axis([3800,2800,0,0.45])
plt.xlabel('Wavenumber (cm-1)').set_fontsize(13)
plt.ylabel('Absorbance').set_fontsize(13)
#ax = fig.gca()
plt.grid()
plt.legend()
#plt.savefig('D:\PhD-WS\Projects\PAC\XP_1-1\DATA\{0}\Plots\DR1\DR1_{0}_All_scans.png'.format(date))
plt.show()
5
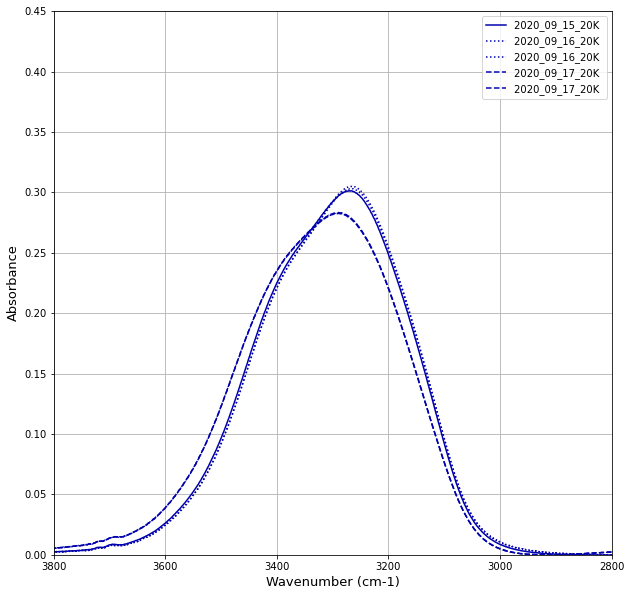
Max Absorbance¶
Import code from DR
Other plotting¶
# imports
%matplotlib inline
from ipywidgets import interactive
import pandas as pd
import numpy as np
# from jupyterthemes import jtplot
# Sample data
np.random.seed(123)
rows = 50
dfx = pd.DataFrame(np.random.randint(90,110,size=(rows, 1)), columns=['Variable X'])
dfy = pd.DataFrame(np.random.randint(25,68,size=(rows, 1)), columns=['Variable Y'])
dfz = pd.DataFrame(np.random.randint(60,70,size=(rows, 1)), columns=['Variable Z'])
df = pd.concat([dfx,dfy,dfz], axis = 1)
#jtplot.style()
import ipywidgets as widgets
from IPython.display import display
def multiplot(a):
opts = df.columns.values
df.loc[:, a].plot()
interactive_plot = interactive(multiplot, a=['Variable X', 'Variable Y', 'Variable Z'])
output = interactive_plot.children[-1]
output.layout.height = '350px'
interactive_plot
Create class with widget input as variable¶
Embed Naming function
class XP_Param:
def found(self):
print("input is" + self.column + self.row )
return XP_Ramp_df_I.loc[self.row,self.column]
T1 = XP_Param()
T1.column = "60K"
T1.row = "2020_09_21"
T1.found()
input is60K2020_09_21
'[6, 7, 8]'
Plotting¶
Data from Data_Annex¶
Data from DR2¶
Interactive Plotting¶
x = numpy.linspace(0, 2 * numpy.pi, 100)
fig, ax = plt.subplots()
line, = ax.plot(x, numpy.sin(x))
ax.grid(True)
def update(change):
line.set_ydata(numpy.sin(change.new * x))
fig.canvas.draw()
int_slider = widgets.IntSlider(
value=1,
min=0, max=10, step=1,
description='$\omega$',
continuous_update=False
)
int_slider.observe(update, 'value')
int_slider
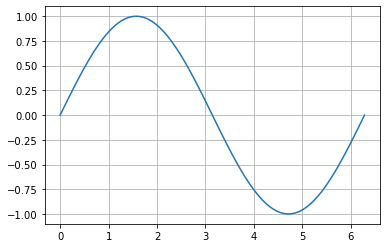
wx GUI creation¶
import wx
# Création d'un nouveau cadre, dérivé du wxPython 'Frame'.
class TestFrame(wx.Frame):
def __init__(self, parent, ID, title):
wx.Frame.__init__(self, parent, -1, title, pos=(0, 0), size=(500, 200))
# À l'intérieur du cadre, créer un panneau..
panel = wx.Panel(self, -1)
# Créer un texte dans le panneau
texte = wx.StaticText(panel, -1, "Bonjour tout le monde!", wx.Point(10, 5), wx.Size(-1, -1))
# Créer un bouton dans le panneau
bouton = wx.Button(panel, -1, "Cliquez-moi!", wx.Point(10, 35), wx.Size(-1, -1))
# lier le bouton à une fonction:
self.Bind(wx.EVT_BUTTON, self.creerDiag, bouton)
# fonction qui affiche une boîte de dialogue
def creerDiag(self, event):
dlg = wx.MessageDialog(self, "Merci de m'avoir cliqué, ça fait du bien.",
"Merci!", wx.ICON_EXCLAMATION | wx.YES_NO | wx.CANCEL)
dlg.ShowModal()
dlg.Destroy()
# Chaque application wxWidgets doit avoir une classe dérivée de wx.App
class TestApp(wx.App):
def OnInit(self):
frame = TestFrame(None, -1, "Test")
self.SetTopWindow(frame)
frame.Show(True)
return True
if __name__ == '__main__':
app = TestApp(0) # créer une nouvelle instance de l'application
app.MainLoop() # lancer l'application
app = wx.App()
frame = wx.Frame(None, title='Simple application')
frame.Show()
app.MainLoop()
---------------------------------------------------------------------------
PyNoAppError Traceback (most recent call last)
<ipython-input-61-95e17b317317> in <module>
1 app = wx.App()
2
----> 3 frame = wx.Frame(None, title='Simple application')
4 frame.Show()
5
PyNoAppError: The wx.App object must be created first!
#!/usr/bin/env python
"""
ZetCode wxPython tutorial
In this example, we create a submenu and a menu
separator.
author: Jan Bodnar
website: www.zetcode.com
last modified: July 2020
"""
import wx
class Example(wx.Frame):
def __init__(self, *args, **kwargs):
super(Example, self).__init__(*args, **kwargs)
self.InitUI()
def InitUI(self):
menubar = wx.MenuBar()
fileMenu = wx.Menu()
fileMenu.Append(wx.ID_NEW, '&New')
fileMenu.Append(wx.ID_OPEN, '&Open')
fileMenu.Append(wx.ID_SAVE, '&Save')
fileMenu.AppendSeparator()
imp = wx.Menu()
imp.Append(wx.ID_ANY, 'Import newsfeed list...')
imp.Append(wx.ID_ANY, 'Import bookmarks...')
imp.Append(wx.ID_ANY, 'Import mail...')
fileMenu.AppendMenu(wx.ID_ANY, 'I&mport', imp)
qmi = wx.MenuItem(fileMenu, wx.ID_EXIT, '&Quit\tCtrl+W')
fileMenu.AppendItem(qmi)
self.Bind(wx.EVT_MENU, self.OnQuit, qmi)
menubar.Append(fileMenu, '&File')
self.SetMenuBar(menubar)
self.SetSize((900, 600))
self.SetTitle('Data Analysis Plotting GUI')
self.Centre()
def OnQuit(self, e):
self.Close()
def main():
app = wx.App()
ex = Example(None)
ex.Show()
app.MainLoop()
if __name__ == '__main__':
main()
<ipython-input-62-2cf284cf38ca>:39: wxPyDeprecationWarning: Call to deprecated item. Use Append instead.
fileMenu.AppendMenu(wx.ID_ANY, 'I&mport', imp)
<ipython-input-62-2cf284cf38ca>:42: wxPyDeprecationWarning: Call to deprecated item. Use Append instead.
fileMenu.AppendItem(qmi)
#!/usr/bin/env python
"""
ZetCode wxPython tutorial
In this example we create a Go To class
layout with wx.BoxSizer.
author: Jan Bodnar
website: www.zetcode.com
last modified: July 2020
"""
import wx
class Example(wx.Frame):
def __init__(self, parent, title):
super(Example, self).__init__(parent, title=title)
self.InitUI()
self.Centre()
def InitUI(self):
panel = wx.Panel(self)
font = wx.SystemSettings.GetFont(wx.SYS_SYSTEM_FONT)
font.SetPointSize(9)
vbox = wx.BoxSizer(wx.VERTICAL)
hbox1 = wx.BoxSizer(wx.HORIZONTAL)
st1 = wx.StaticText(panel, label='Class Name')
st1.SetFont(font)
hbox1.Add(st1, flag=wx.RIGHT, border=8)
tc = wx.TextCtrl(panel)
hbox1.Add(tc, proportion=1)
vbox.Add(hbox1, flag=wx.EXPAND|wx.LEFT|wx.RIGHT|wx.TOP, border=10)
vbox.Add((-1, 10))
hbox2 = wx.BoxSizer(wx.HORIZONTAL)
st2 = wx.StaticText(panel, label='Matching Classes')
st2.SetFont(font)
hbox2.Add(st2)
vbox.Add(hbox2, flag=wx.LEFT | wx.TOP, border=10)
vbox.Add((-1, 10))
hbox3 = wx.BoxSizer(wx.HORIZONTAL)
tc2 = wx.TextCtrl(panel, style=wx.TE_MULTILINE)
hbox3.Add(tc2, proportion=1, flag=wx.EXPAND)
vbox.Add(hbox3, proportion=1, flag=wx.LEFT|wx.RIGHT|wx.EXPAND,
border=10)
vbox.Add((-1, 25))
hbox4 = wx.BoxSizer(wx.HORIZONTAL)
cb1 = wx.CheckBox(panel, label='Case Sensitive')
cb1.SetFont(font)
hbox4.Add(cb1)
cb2 = wx.CheckBox(panel, label='Nested Classes')
cb2.SetFont(font)
hbox4.Add(cb2, flag=wx.LEFT, border=10)
cb3 = wx.CheckBox(panel, label='Non-Project classes')
cb3.SetFont(font)
hbox4.Add(cb3, flag=wx.LEFT, border=10)
vbox.Add(hbox4, flag=wx.LEFT, border=10)
vbox.Add((-1, 25))
hbox5 = wx.BoxSizer(wx.HORIZONTAL)
btn1 = wx.Button(panel, label='Ok', size=(70, 30))
hbox5.Add(btn1)
btn2 = wx.Button(panel, label='Close', size=(70, 30))
hbox5.Add(btn2, flag=wx.LEFT|wx.BOTTOM, border=5)
vbox.Add(hbox5, flag=wx.ALIGN_RIGHT|wx.RIGHT, border=10)
panel.SetSizer(vbox)
def main():
app = wx.App()
ex = Example(None, title='Go To Class')
ex.Show()
app.MainLoop()
if __name__ == '__main__':
main()
%load_ext watermark
---------------------------------------------------------------------------
ModuleNotFoundError Traceback (most recent call last)
<ipython-input-63-a0d979a7c178> in <module>
----> 1 get_ipython().run_line_magic('load_ext', 'watermark')
~\Anaconda3\lib\site-packages\IPython\core\interactiveshell.py in run_line_magic(self, magic_name, line, _stack_depth)
2324 kwargs['local_ns'] = sys._getframe(stack_depth).f_locals
2325 with self.builtin_trap:
-> 2326 result = fn(*args, **kwargs)
2327 return result
2328
<decorator-gen-65> in load_ext(self, module_str)
~\Anaconda3\lib\site-packages\IPython\core\magic.py in <lambda>(f, *a, **k)
185 # but it's overkill for just that one bit of state.
186 def magic_deco(arg):
--> 187 call = lambda f, *a, **k: f(*a, **k)
188
189 if callable(arg):
~\Anaconda3\lib\site-packages\IPython\core\magics\extension.py in load_ext(self, module_str)
31 if not module_str:
32 raise UsageError('Missing module name.')
---> 33 res = self.shell.extension_manager.load_extension(module_str)
34
35 if res == 'already loaded':
~\Anaconda3\lib\site-packages\IPython\core\extensions.py in load_extension(self, module_str)
78 if module_str not in sys.modules:
79 with prepended_to_syspath(self.ipython_extension_dir):
---> 80 mod = import_module(module_str)
81 if mod.__file__.startswith(self.ipython_extension_dir):
82 print(("Loading extensions from {dir} is deprecated. "
~\Anaconda3\lib\importlib\__init__.py in import_module(name, package)
125 break
126 level += 1
--> 127 return _bootstrap._gcd_import(name[level:], package, level)
128
129
~\Anaconda3\lib\importlib\_bootstrap.py in _gcd_import(name, package, level)
~\Anaconda3\lib\importlib\_bootstrap.py in _find_and_load(name, import_)
~\Anaconda3\lib\importlib\_bootstrap.py in _find_and_load_unlocked(name, import_)
ModuleNotFoundError: No module named 'watermark'
Package version for binder¶
%watermark --iversions
matplotlib: 3.2.2
ipywidgets: 7.6.3
re : 2.2.1
wx : 4.1.1
pandas : 1.0.5
numpy : 1.18.5